SwiftUI ScrollView
What is ScrollView
ScrollView
is a view that allows the scrolling of its contained views.
We use this view when we have a large content view that can't be present within a device screen.
In this example, we use s scroll view to present a long text list.
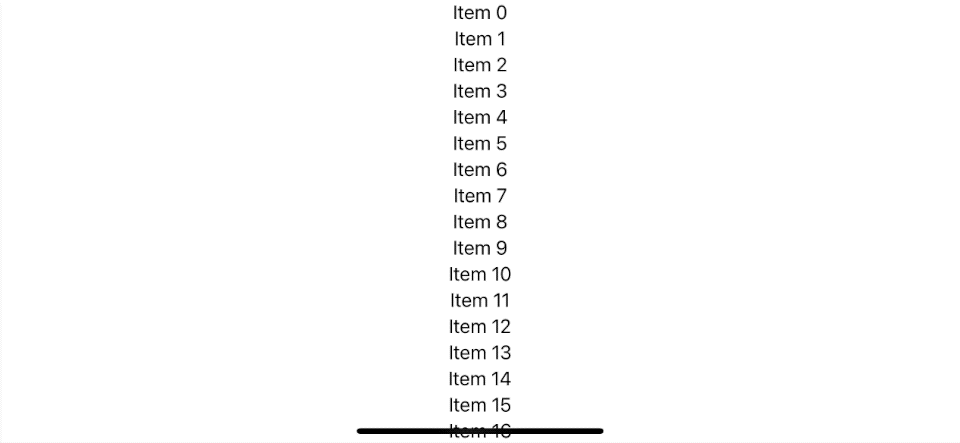
How to use SwiftUI ScrollView
To use ScrollView
, you put a content view that you want to make scrollable as a scroll view content.
struct ScrollViewDemo: View {
var body: some View {
ScrollView {
VStack {
ForEach(0..<100) { i in
Text("Item \(i)")
}
}.frame(maxWidth: .infinity)
}
}
}
ScrollView direction
By default, ScrollView
make content scrollable on a vertical axis. You can override this by providing Axis.Set
when initializing a scroll view.
You have three options to set a support scrolling axes.
- Vertical:
ScrollView
orScrollView(.vertical)
- Horizontal:
ScrollView(.horizontal)
- Both vertical and horizontal:
ScrollView([.horizontal, .vertical])
Beware that scrollable axes only set the direction of a scroll view, not the content.
In this example, we set the scrollable axis to .horizontal
while the content is still rendered vertically in VStack
.
struct ScrollViewDemo: View {
var body: some View {
ScrollView(.horizontal) {
VStack {
ForEach(0..<100) { i in
Text("Item \(i)")
}
}.frame(maxWidth: .infinity)
}
}
}
This ScrollView
can only scroll horizontally, but the content renders vertically and goes offscreen.
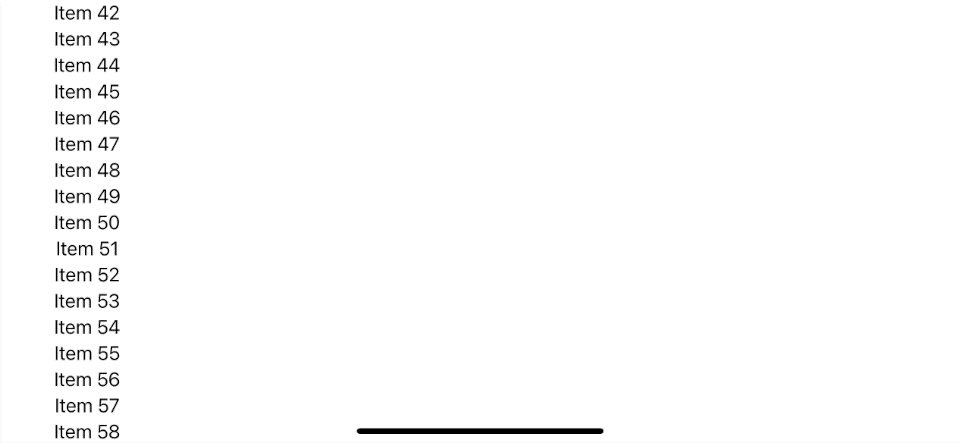
If you want to scroll horizontally, you need to change the layout of your content too.
Here is an example of horizontal scroll view with HStack
content.
struct ScrollViewDemo: View {
var body: some View {
ScrollView(.horizontal) {
HStack {
ForEach(0..<100) { i in
Text("Item \(i)")
}
}.frame(maxHeight: .infinity)
}
}
}
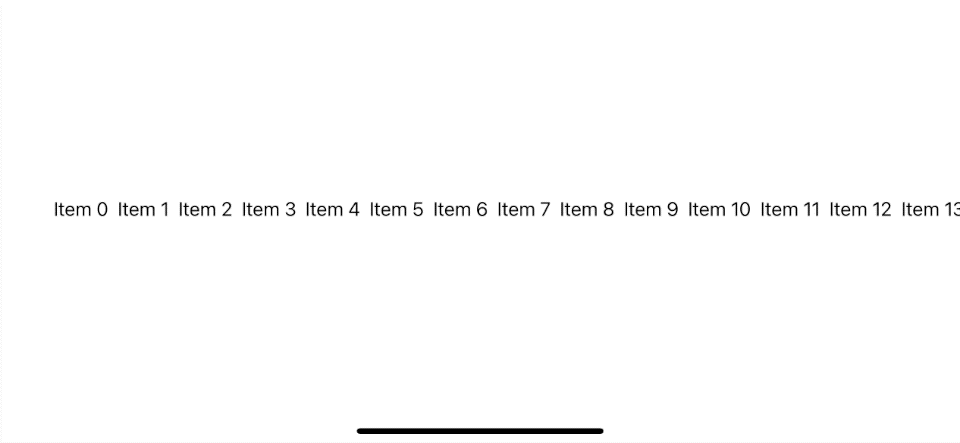